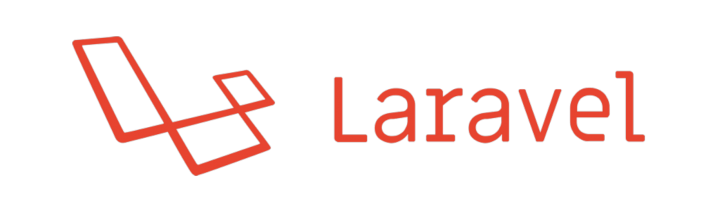
Sending notifications in Laravel is quite easy actually.
As you may know that Laravel notification system is a beauty on its own. It’s easy to follow up and learn. But it’s often the case that documentation is not enough (as in many things that laravel offers), so let’s dive in.
In order, we will be doing these steps;
- Creating an Event
- Creating a Listener for that Event
- Creating a Notification to be sent with that Listener
- Sending the Notification by firing the event
Imagine that you are building an e-commerce site, it’s common to receive an order notification when an order has been placed. We will be using email channel for the notification.
CREATING EVENT
Let’s start with creating our event, name it as OrderCreatedEvent;
php artisan make:event OrderCreatedEvent
This will create the class below;
<?php
namespace App\Events;
use Illuminate\Broadcasting\Channel;
use Illuminate\Broadcasting\InteractsWithSockets;
use Illuminate\Broadcasting\PresenceChannel;
use Illuminate\Broadcasting\PrivateChannel;
use Illuminate\Contracts\Broadcasting\ShouldBroadcast;
use Illuminate\Foundation\Events\Dispatchable;
use Illuminate\Queue\SerializesModels;
class OrderCreatedEvent
{
use Dispatchable, InteractsWithSockets, SerializesModels;
public $user;
/**
* Create a new event instance.
*
* @param $user
*/
public function __construct($user)
{
$this->user = $user;
}
/**
* Get the channels the event should broadcast on.
*
* @return Channel|array
*/
public function broadcastOn()
{
return new PrivateChannel('channel-name');
}
}
Be aware that I’m passing the user in constructor, so whenever this event fired we need to give it a User model instance.
CREATING LISTENER
Now create our listener, name it as;
php artisan make:listener OrderCreatedListener
We need to pass the OrderCreatedEvent class to the OrderCreatedListener handle method.
<?php
namespace App\Listeners;
use App\Events\OrderCreatedEvent ;
use Illuminate\Support\Facades\Notification;
class OrderCreatedListener
{
/**
* Create the event listener.
*
* @param void
*/
public function __construct()
{
}
/**
* Handle the event.
*
* @param OrderCreatedEvent $event
* @return void
*/
public function handle(OrderCreatedEvent $event)
{
}
}
CREATING A NOTIFICATION
Next step is creating our notification;
php artisan make:notification OrderCreatedNotification
Make sure its content to be same as below;
<?php
namespace App\Notifications;
use Illuminate\Bus\Queueable;
use Illuminate\Contracts\Queue\ShouldQueue;
use Illuminate\Notifications\Messages\MailMessage;
use Illuminate\Notifications\Notification;
class OrderCreatedNotification extends Notification implements ShouldQueue
{
use Queueable;
private $user;
/**
* Create a new notification instance.
*
* @param $user
*/
public function __construct($user)
{
$this->user = $user;
$this->delay(now()->addSeconds(5));
}
/**
* Get the notification's delivery channels.
*
* @param mixed $notifiable
* @return array
*/
public function via($notifiable)
{
return ['mail'];
}
/**
* Get the mail representation of the notification.
*
* @param mixed $notifiable
* @return \Illuminate\Notifications\Messages\MailMessage
*/
public function toMail($notifiable)
{
return (new MailMessage)
->line('Your order placed on our store.')
->action('Notification Action', url('/'))
->line('You can see your order details below!');
}
/**
* Get the array representation of the notification.
*
* @param mixed $notifiable
* @return array
*/
public function toArray($notifiable)
{
return [
//
];
}
}
Notice the delay and user param in constructor. The listener we just created passing a user param to our notification and we are adding delay for 5 seconds. Also notice the “implements” “ShouldQueue” contract, meaning this notification will be in queue, so make sure to edit .env credentials about queue.
We need to edit our Listener as;
<?php
namespace App\Listeners;
use App\Events\OrderCreatedEvent ;
use App\Notifications\OrderCreatedNotification;
use Illuminate\Support\Facades\Notification;
class OrderCreatedListener
{
/**
* Create the event listener.
*
* @param void
*/
public function __construct()
{
}
/**
* Handle the event.
*
* @param OrderCreatedEvent $event
* @return void
*/
public function handle(OrderCreatedEvent $event)
{
Notification::send($event->user, new OrderCreatedNotification($event->user)); // this line is responsible for sending the notification
// $event->notify() // would also work with proper signature
}
}
Take a look at the handle method now. Above I’m using Notification facade to send the notification, but you could also choose to send the notification with “$event->notify()” method. Both will work.
ADDING EVENT AND LISTENER TO EVENT SERVICE PROVIDER
Now we should add the event and listener to the laravel provided EventServiceProvider.
class EventServiceProvider extends ServiceProvider
{
/**
* The event listener mappings for the application.
*
* @var array
*/
protected $listen = [
OrderCreatedEvent::class => [
OrderCreatedListener::class
]
];
/**
* Register any events for your application.
*
* @return void
*/
public function boot()
{
//
}
}
Now don’t forget to run your queue;
php artisan queue:work
FIRING THE EVENT
Last step is firing the event somewhere in our application, also don’t forget to pass the user parameter;
event(new OrderCreatedEvent($user));
Test it now, depending on your queue configuration your notification will be sent.